Hash Practice
This script picks a random password, hashes it with SHA-256 or MD5, and saves the hash to a file on your desktop. You then try to “crack the hash”. It runs in a loop until you type “exit.
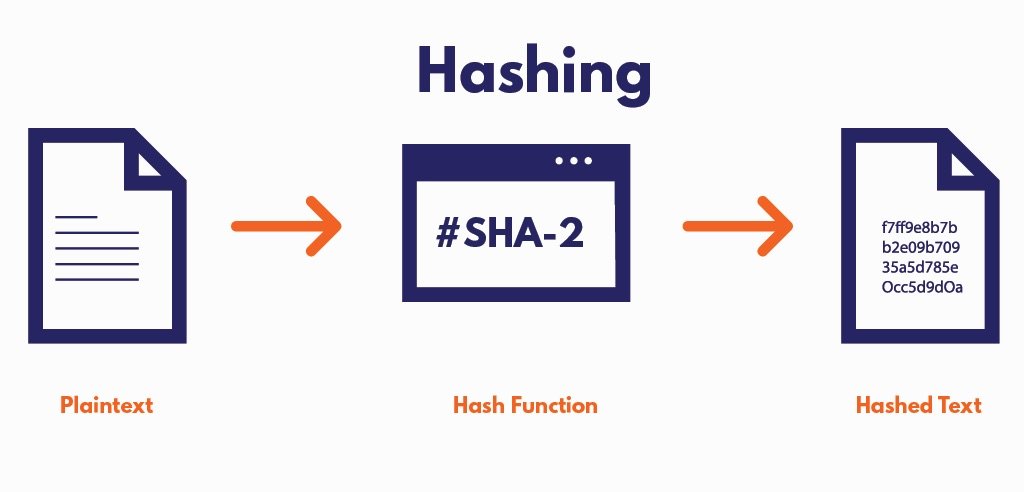
Hash Game
(Python)
Script:
import hashlib
import random
import os
# A list of passwords
given_passwords = [
"password", "123456", "123456789", "12345", "1234", "qwerty", "abc123","password123", "letmein", "welcome", "sunshine", "iloveyou", "princess", "admin", "trustno1", "password1", "Str0ng!Passw0rd", "QwErTy2024!", "S3cure*Login#", "P@ssw0rd!987", "Admin@1234", "Th1sIs$Crazy", "LetM3!nPlz", "Summer#2023", "W1nt3r$Chill", "B3tterThanBefore!", "Pa$$wordCr@zy", "ZxC!vBnM987", "N0t4Common1!", "!SecureMeNow!", "Y0uCantGuessTh1s", "F@stN3tC0nn!", "123Go!Secure", "Alpha$Beta#2025", "Try2H@ckTh1s!", "Hidden#Truth42", "C0ffee#Beanz!", "MyD0g$N@meIsRex", "Wh0AteMyT@co!", "G00dLuck#123", "Ninja!2025^", "R@inB0w_Un1c0rn", "5tarD*st999", "BaseB@ll!Rules", "M0nkeyKing#", "Ski&Board_321", "Never@GuessMe!", "Il0ve$Python3", "!Dance2Night!", "SecureM3Now!!", "Up&Down123!", "9Lives&Counting", "Qwerty!Zxcvbn", "Chess#Champ2024", "I<3SecurePwd", "L0ck&L0ad!", "Sp@ce$Force42", "W@rriorPrincess", "T1m3Tr@vel!99", "Ctrl#Alt#Win", "Pizza!Lover#8", "Superman!123", "Football@321", "Hello123!q", "Princess!99", "Batman2024!@", "MonkeyKing#42", "StarWars!2025", "TrustNo1#123", "Qwerty!Pass1", "Admin#2024!", "Welcome@1234", "Passw0rd!4Life", "LetMeIn!2024", "Secure$Pass123", "M0nkey!Rocks", "Master@Cr@ck", "Password1@Safe", "Freed0m!Pass", "Summer@12345", "Shadow2025!@", "Ninja!Xtreme44", "Power@12345", "H@cker12345!", "GameOn!Qwerty", "Login$Pass123", "StrongPassw0rd#", "M1chelL@93", "Hello!Rock123", "CrackM3Up!2024", "JustDoIt$19", "SummerFun!2025", "Password2!Free", "Winter@Snow2024", "Qwerty$Master", "Football@Win9", "Let$MeIn@Secure", "XxX@J0rdanXxX", "M0nkeyKing$@123", "Adm1n@123456", "No!MorePass$", "H@ckM3@2025", "Warrior!Rocks123", "Purple!Pass2024", "Dragon!Fire@55", "NewDay!2023#", "N0t4Guess!True", "P@ssw0rd!2023", "Hidden#Truth!1", "Future!Pass#321", "Happy@12345", "starwars", "dragon", "monkey", "123123", "football", "iloveu", "123qwe", "shadow", "master","superman", "1qaz2wsx", "qwerty123", "baseball", "michael", "111111", "freedom", "chocolate", "tigger", "jessica", "secret", "password12", "dragon1234", "123password", "qwerty789", "princess1", "abcdef", "monkeyking", "tiger", "qwerty1", "iloveyou123", "qwertyuiop", "qwerty1q2", "1qazxsw23", "admin1", "shadow1", "password11", "superman123", "letmein12345", "maggie", "letmein123", "1q2w3e4r5t", "1234abcd", "123qwerty", "sunshine123", "freedom123", "master123", "696969", "pepper", "ashley", "nicole", "chelsea", "biteme", "passw0rd", "bailey", "hannah", "michael1"
]
def hash_sha256(text):
return hashlib.sha256(text.encode()).hexdigest()
def hash_md5(text):
return hashlib.md5(text.encode()).hexdigest()
def write_hash_to_file(hash_value):
desktop = os.path.join(os.path.expanduser("~"), "Desktop")
file_path = os.path.join(desktop, "hashed_password.txt")
try:
with open(file_path, 'w') as file:
file.write(hash_value + '\n')
except IOError:
print("Something went wrong writing to the file.")
def main():
print("== Hash Guessing Game ==")
print("You'll be shown a hash. Try to guess the original password.")
print("Hash will be saved to a file on your Desktop.")
print("\nSelect hash type:")
print("1) SHA-256")
print("2) MD5")
choice = input("Your choice (1 or 2): ").strip()
if choice not in {'1', '2'}:
print("Not a valid choice. Exiting...")
return
while True:
original_password = random.choice(given_passwords)
hash_fn = hash_sha256 if choice == '1' else hash_md5
hash_result = hash_fn(original_password)
write_hash_to_file(hash_result)
print("\nA hash has been written to 'hashed_password.txt' on your Desktop.")
user_input = input("Enter your guess (or type 'exit' to quit): ").strip()
if user_input.lower() == 'exit':
print("Thanks for playing. Goodbye!")
break
if user_input == original_password:
print("Nice! You got it right.")
else:
print("Nope, that's not it. Try again!")
if __name__ == "__main__":
main()